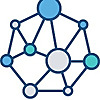
Algorithms, Blockchain and Cloud
2 FOLLOWERS
Algorithms, Blockchain, and Cloud are the Ultimate Computer Technology Blogs. The blog provides useful and well-written articles related to computing, programming, algorithms, data structures, and online tools. Algorithms, Blockchain, and Cloud
Algorithms, Blockchain and Cloud
1d ago
This post writes a go version of caching layer using redis, wrapps it in a class and provides a usage. Let’s create a Go package that provides a caching layer using Redis. We’ll use the popular go-redis/redis/v8 package to interact with Redis. We’ll define a Cache struct with methods to set and get values, and wrap the functionality in a class-like structure. First, you need to install the go-redis/redis/v8 package: go get github.com/go-redis/redis/v8 Redis Cache Layer in Go Now, let’s create our caching layer in Go. package cache import ( "context" "time" "github.com/go-redis/redis/v8" ) // C ..read more
Algorithms, Blockchain and Cloud
1d ago
Teaching Kids Programming: Videos on Data Structures and Algorithms Implement the Pairwise Function in Python using the Iterator/Yield The pairwise is from itertools where we can iterate over a iterable (list/array, tuple, set, dictionary, string and etc) in Python, and then returns an iterator to the tuple of the adjacent elements. For example: from itertools import pairwise s = "1234567" for a, b in pairwise(s): print(a, b) This prints the following: 1, 2 2, 3 3, 4 4, 5 5, 6 6, 7 This is a handy syntax sugar to avoid dealing with the index for example, you can do Continue Reading »
The post ..read more
Algorithms, Blockchain and Cloud
2d ago
Recently, I have seen my servers overloaded (High CPU Usage) and I looked into the apache logs and I see the access logs from ChatGPT Bot aka GPTBot/1.0 and Bytedance Bots aka Bytespider. You can check the top 10 IPs that access your server via the following BASH command: #!/bin/bash awk '{a++}END{for(v in a)print v, a}' /var/log/apache2/*.log* | sort -k2 -nr | head -10 Why You Should Stop the ChatGPT and Bytedance Bots Crawling Your Pages? These bots are using your materials (information or data) for free. Their bots crawl your data and then use them to train their LLMs Continue Reading »
The ..read more
Algorithms, Blockchain and Cloud
2d ago
Beware of the Wirex Crypto Phishing Email Scam: I Lost 1000 GBP worth of USDT I have been a victim of a scam yesterday and I lost about 1355 USDT Crypto token (worth of 1000 GBP) due to opening a phishing email on the phone and had my phone number “verified”. I was doing some housework, and I received this phishing email see below. It looked so real on the Apple iPhone Pro 15 Max, which didn’t reveal the actual email addresses. If I had it opened on desktop, I would immediately see it as a scam/fake email. Anyway, I Continue Reading »
The post The Major Security Flaws of Wirex (WirexApp) Excha ..read more
Algorithms, Blockchain and Cloud
1w ago
Teaching Kids Programming: Videos on Data Structures and Algorithms Apple Redistribution into Boxes You are given an array apple of size n and an array capacity of size m. There are n packs where the ith pack contains apple apples. There are m boxes as well, and the ith box has a capacity of capacity apples. Return the minimum number of boxes you need to select to redistribute these n packs of apples into boxes. Note that, apples from the same pack can be distributed into different boxes. Example 1: Input: apple = , capacity = Output: 2 Explanation: Continue Reading »
The post Teaching Kids Pr ..read more
Algorithms, Blockchain and Cloud
2w ago
In Rust programming, Cargo is a package manager and build system. It’s used for managing your Rust project’s dependencies, building your project, and managing its distribution. Cargo relies on two main files to manage these tasks: Cargo.toml and Cargo.lock. What is Cargo.toml? This is the manifest file for your Rust project. It’s written in TOML (Tom’s Obvious, Minimal Language), which is a simple configuration file format. Cargo.toml contains metadata about your project, such as its name, version, authors, dependencies, build configuration, and other project-specific settings. Here’s an examp ..read more
Algorithms, Blockchain and Cloud
2w ago
Teaching Kids Programming: Videos on Data Structures and Algorithms You are given a binary array nums. We call a subarray alternating if no two adjacent elements in the subarray have the same value. Return the number of alternating subarrays in nums. Example 1: Input: nums = Output: 5 Explanation: The following subarrays are alternating: , , , , and . Example 2: Input: nums = Output: 10 Explanation: Every subarray of the array is alternating. There are 10 possible subarrays that we can choose. Constraints: 1 <= nums.length <= 10^5 nums is either 0 or 1. Hints: Try Continue Reading »
The ..read more
Algorithms, Blockchain and Cloud
1M ago
Teaching Kids Programming: Videos on Data Structures and Algorithms Given a string s, find any substring of length 2 which is also present in the reverse of s. Return true if such a substring exists, and false otherwise. Example 1: Input: s = “leetcode” Output: true Explanation: Substring “ee” is of length 2 which is also present in reverse(s) == “edocteel”. Example 2: Input: s = “abcba” Output: true Explanation: All of the substrings of length 2 “ab”, “bc”, “cb”, “ba” are also present in reverse(s) == “abcba”. Example 3: Input: s = “abcd” Output: false Explanation: There is no Continue Readin ..read more
Algorithms, Blockchain and Cloud
1M ago
On Tron Blockchain, a wallet could be inactivated as it doesn’t appear on the chain yet. The way to activate a Tron Wallet is to send a 1 TRX to it, so that the wallet/transaction is recorded on the Tron Blockchain. How to Check if a String is a Valid TRON wallet? We can use the TronWeb to validate if a given string is a valid TRON wallet address (although it can be inactive): // Function to check if a TRON wallet address is valid // param: address to check if it is valid tron wallet // param: tron network Continue Reading »
The post NodeJs Function to Check if a Tron Wallet Address is Valid a ..read more
Algorithms, Blockchain and Cloud
1M ago
Chrome is deprecating the Manifest v2 From June 2024. And this post will show you the steps to convert your extension with Manifest v2 to Manifest v3. Google has given enough time to migrate to v3. The v3 manifest was first introduced in Dec 2020 (Chrome version M88 Beta), and Google has given developers more than 3 years for phasing out v2. Changes required to Convert v2 Manifest to V3 for Chrome Extensions The most changes you need are in the manifest.json: Here are the details required to modify for manifest.json manifest_version This needs to be changed to 3 (from Continue Reading »
The po ..read more