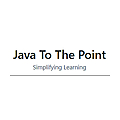
Java To The Point - Simplifying Learning
411 FOLLOWERS
Welcome to our Java website! We are a team of highly skilled developers and experts with a passion for creating innovative solutions using the power of Java. With over a decade of experience in the industry, our team has worked with clients from various sectors, including finance, healthcare, e-commerce, and education. We have a deep understanding of the latest industry trends, technologies,..
Java To The Point - Simplifying Learning
1y ago
In Java, a dictionary is a data structure that stores key-value pairs. The Java API provides the Dictionary class to implement a dictionary. The Dictionary class is an abstract class that defines a data structure for managing key-value pairs. It is the superclass of the Hashtable and Properties classes.
The Dictionary class is a legacy class, which means it was introduced in an earlier version of Java and has been replaced by newer classes. However, it is still supported and used in many legacy applications.
Features of Dictionary Class
The Dictionary class provides methods for adding, removi ..read more
Java To The Point - Simplifying Learning
1y ago
In Java, a HashMap is a popular implementation of the Map interface that allows us to store and retrieve key-value pairs. However, in multi-threaded applications, accessing a HashMap concurrently can lead to race conditions and thread-safety issues. To address this problem, Java provides the ConcurrentHashMap class, which is a thread-safe implementation of the Map interface. In this article, we will take a closer look at what ConcurrentHashMap is and how it works in Java.
What is ConcurrentHashMap?
ConcurrentHashMap is a thread-safe implementation of the Map interface in Java that allows multi ..read more
Java To The Point - Simplifying Learning
1y ago
In Java, a HashMap is a popular implementation of the Map interface that allows us to store and retrieve key-value pairs. However, in some situations, we might need to use an IdentityHashMap instead of a regular HashMap. In this article, we will take a closer look at what an IdentityHashMap is and how it works in Java.
What is an IdentityHashMap?
An IdentityHashMap is a special implementation of the Map interface in Java that uses the reference equality operator (==) instead of the equals() method to compare keys. This means that keys are considered equal if and only if they refer to the same ..read more
Java To The Point - Simplifying Learning
1y ago
A LinkedHashMap in Java is a specialized implementation of the Map interface that maintains a linked list of the entries in the map, in the order in which they were inserted. In this article, we will take a closer look at how LinkedHashMap works in Java.
What is a LinkedHashMap?
A LinkedHashMap is a subclass of the java.util.HashMap class that provides a way to store key-value pairs in a way that maintains their insertion order. Each key-value pair in a LinkedHashMap is stored as an Entry object that contains a reference to the key, the value, and pointers to the next and previous entries in t ..read more
Java To The Point - Simplifying Learning
1y ago
A WeakHashMap in Java is a special implementation of the Map interface that provides a way to store key-value pairs in a way that allows the keys to be garbage-collected if they are not being used anymore. In this article, we will take a closer look at how WeakHashMap works in Java.
What is a WeakHashMap?
A WeakHashMap is a subclass of the java.util.AbstractMap class that provides a way to store key-value pairs in a way that allows the keys to be garbage-collected if they are no longer being used.
In a regular HashMap, once a key is added to the map, it remains in memory as long as the map exi ..read more
Java To The Point - Simplifying Learning
1y ago
HashMap is one of the most frequently used collections in Java. It provides an efficient way to store and retrieve data in a key-value format. In this article, we will take a closer look at how HashMap works internally in Java.
Understanding Hashing
Before we dive into how HashMap works, we need to understand the concept of hashing. Hashing is the process of mapping a large data set of variable size to a smaller data set of a fixed size. In the case of HashMap, it involves mapping keys to indices in an underlying array.
Hash Function
The first step in using a HashMap is to define a hash functi ..read more
Java To The Point - Simplifying Learning
1y ago
A HashMap in Java is a collection that stores data in key-value pairs. It is one of the most commonly used data structures in Java, and it provides an efficient way to store and retrieve data. In this article, we will explore HashMap in Java, how it works, and some common use cases.
HashMap in Java
A HashMap is a part of the Java Collections framework and is used to store data in key-value pairs. It is implemented using an array of linked lists and provides constant-time performance for basic operations such as adding, removing, and retrieving elements.
The HashMap class in Java is generic, wh ..read more
Java To The Point - Simplifying Learning
1y ago
Java 17, also known as JDK 17, is the latest release of the Java Development Kit, and was released on September 14, 2021. It is a long-term support (LTS) release, meaning it will receive support and updates for a minimum of eight years. Java 17 includes a variety of new features, improvements, and bug fixes that make it a significant release for developers.
New Features in Java 17
Some of the new features and enhancements in Java 17 include:
Sealed Classes: This feature allows developers to restrict the set of classes that can extend or implement a class or interface. Sealed classes provide m ..read more
Java To The Point - Simplifying Learning
1y ago
Java 11, also known as JDK 11, was released on September 25th, 2018 as a long-term support (LTS) release of the Java Development Kit. It was the first LTS release since Java 8, which was released in 2014. Java 11 includes a number of new features, performance improvements, and security enhancements.
New Features in Java 11
Some of the new features introduced in Java 11 include:
Local-Variable Syntax for Lambda Parameters: This feature allows you to declare the type of a lambda expression’s parameter using the var keyword, similar to how you can declare the type of a local variable.
HTTP Clien ..read more
Java To The Point - Simplifying Learning
1y ago
In Java, EnumMap is a specialized implementation of the Map interface that is designed for use with enum keys. It provides a high-performance and type-safe implementation of a map that is specifically tailored to work with enum keys. This makes it a useful tool for any application that requires the use of enums as keys in a map.
EnumMap in Java
The EnumMap class in Java was introduced in JDK 5 as part of the Java Collections Framework. It is a specialized implementation of the Map interface that is designed to work specifically with enum keys. Unlike a regular HashMap or TreeMap, which can us ..read more