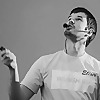
Sobolevn’s Personal Blog
25 FOLLOWERS
I am Nikita Sobolev. I work as a CTO at wemake.services, I also happen to be a founder of this company. I also run a few side projects. I work with python, elixir, and javascript (typescript too). But, I am also familiar with many other languages.
Sobolevn’s Personal Blog
1y ago
Before ParamSpec (PEP612) was released in Python3.10 and typing_extensions, there was a big problem in typing decorators that change a function’s signature.
Let’s start with a basic example. How one can type a decorator function that does not change anything?
from typing import Callable, TypeVar
C = TypeVar('C', bound=Callable)
def logger(function: C) -> C:
def decorator(*args, **kwargs):
print('Function called!')
return function(*args, **kwargs)
return decorator
Notice the most important part here: C = TypeVar('C', bound=Callable)
What does it mean? It means th ..read more
Sobolevn’s Personal Blog
1y ago
Today I am going to introduce a new concept for Python developers: typeclasses. It is a concept behind our new dry-python library called classes.
I will tell you in advance, that it will look very familiar to what you already know and possibly even use. Moreover, we reuse a lot of existing code from Python’s standard library. So, you can call this approach “native” and “pythonic”. And it is still going to be interesting: I am showing examples in 4 different languages!
But, before discussing typeclasses themselves, let’s discuss what problem they do solve.
Some functions must behave differently ..read more
Sobolevn’s Personal Blog
1y ago
Today I am going to discuss quite a new idea for Python users, an idea of making tests a valuable part of your application.
Let’s jump into it.
Current status
Right now the status-quo for source code/tests dualism is that you ship source code to your library users and most often do not include your tests in any manner.
Sometimes people also attach the tests/ folder to a release, so they are just laying around just in case. Most of the time they are useless to the end-user.
And what is the most important part of it all is that our users are often find themselves in a situation when they have t ..read more
Sobolevn’s Personal Blog
1y ago
dry-python/returns@0.15 is released! And it means that now anyone can use our Higher Kinded Types emulation in their projects.
In this post I will explain:
What Higher Kinded Types (HKTs) are and why they are useful
How they are implemented and what limitations there are
How can you use them in your own projects
Without further ado, let’s talk about typing!
Simple types
Typing is layered. Like a good cake. There are at least three layers that we are going to cover.
Simple (or flat) typing, like x: int = 1. This allows us to express simple types and their transformations. Like int -> str ..read more
Sobolevn’s Personal Blog
1y ago
In the last few years async keyword and semantics made its way into many popular programming languages: JavaScript, Rust, C#, and many others languages that I don’t know or don’t use.
Of course, Python also has async and await keywords since python3.5.
In this article, I would like to provide my opinion about this feature, think of alternatives, and provide a new solution.
Colours of functions
When introducing async functions into the languages, we actually end up with a split world. Now, some functions start to be red (or async) and old ones continue to be blue (sync).
The thing about this di ..read more
Sobolevn’s Personal Blog
1y ago
Almost every week I accidentally get into this logging argument. Here’s the problem: people tend to log different things and call it a best-practice. And I am not sure why. When I start discussing this with other people I always end up repeating the exact same ideas over and over again.
So. Today I want to criticize the whole logging culture and provide a bunch of alternatives.
Logging does not make sense
Let’s start with the most important one. Logging does not make any sense!
Let’s review a popular code sample that you can find all across the internet:
try:
do_something_complex(*args ..read more
Sobolevn’s Personal Blog
1y ago
Recently I had to add python3.8 for our Python linter (the strictest one in existence): wemake-python-styleguide. And during this straight-forward (at first look) task, I have found several problems with test coverage that were not solved in Python community at all.
Let’s dig into it.
Environment-based logic
The thing about this update was that python3.8 introduced a new API. Previously we had visit_Num, visit_Str, visit_NameConstant methods (we don’t really care about what they do, only names are important), but now we have to use visit_Constant method instead.
Well, we will have to write so ..read more
Sobolevn’s Personal Blog
1y ago
Dependency injection is a controversial topic. There are known problems, hacks, and even whole methodologies on how to work with DI frameworks.
A lot of people asked me: how can you use a lot of typed functional concepts together with traditional object-oriented dependency injection?
And this question makes a lot of sense. Because functional programming is all about composition. And dependency injection is just magic. You get some almost random objects injected into some places of your code. Moreover, the whole container to be injected later is magically assembled from the separate parts at r ..read more
Sobolevn’s Personal Blog
1y ago
Dear internet,
Today we have screwed up by applying a broken migration to the running production service and causing a massive outage for several hours… Because the rollback function was terribly broken as well.
As a result, we had to restore a backup that was made several hours ago, losing some new data.
Why did it happen?
The easiest answer is just to say: “Because it is X’s fault! He is the author of this migration, he should learn how databases work”. But, it is counterproductive.
Instead, as a part of our “Blameless environment” culture, we tend to put all the guilt on the CI. It was the ..read more
Sobolevn’s Personal Blog
1y ago
When talking about “bad code” people almost certainly mean “complex code” among other popular problems. The thing about complexity is that it comes out of nowhere. You start your fairly simple project, then one day you find it in ruins. And no one knows how and when it happened.
But, this ultimately happens for a reason! Code complexity enters your codebase in two possible ways: with big chunks and incremental additions. And people are bad at reviewing and finding both of them.
When a big chunk of code comes in, the reviewer will be challenged to find the exact location where the code is comp ..read more