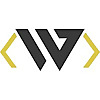
Wanago » TypeScript
112 FOLLOWERS
Wanago features blog series like TypeScript Express Tutorial, API with NestJS, JavaScript design patterns, and more useful articles. Wanago is a JavaScript blog focused on covering practical issues and explaining how the language works under the surface.
Wanago » TypeScript
1M ago
When a NestJS application starts, it creates instances of various classes, such as controllers and services. By default, NestJS treats those classes as singletons, where a particular class has only one instance. NestJS then shares the single instance of each provider across the entire application’s lifetime, creating a singleton provider scope.
If you want to know more about the Singleton pattern, check out JavaScript design patterns #1. Singleton and the Module
The singleton scope fits most use cases. Thanks to creating just one instance of each provider and sharing it with all consumers, N ..read more
Wanago » TypeScript
2M ago
Managing money is a part of developing web applications that we can’t cut corners on. This article explores various data types built into PostgreSQL and Prisma that we could use to handle finances. We also discuss which ones to avoid and why.
Why we should avoid floating point numbers
We are used to using fractions when dealing with money – for example, $15.20 means fifteen dollars and twenty cents.
Therefore, we might think of using floating-point types to store the monetary values. Using floating-point numbers is a very popular way to represent numbers that include a fraction.
schema.pr ..read more
Wanago » TypeScript
2M ago
Often, we might have a situation where a single entity, such as a comment, needs to be associated with more than one type of table. For example, the user might be able to comment on articles, photos, and more. One solution would be to create a separate table for each type of comment, such as ArticleComment and PhotoComment. This could lead to duplicating a lot of logic since a comment for an article or a photo would contain the same columns, such as the author and the content.
An alternative would be to implement polymorphic association. It is a design pattern where a table can be associa ..read more
Wanago » TypeScript
2M ago
Ensuring that our application is secure is one of the most important things we must do as developers. One of the ways to protect our application from well-known vulnerabilities is to set appropriate response headers. There are quite a lot of different security-related response headers to consider. Fortunately, the helmet library can set them for us.
Helmet maintains a set of various response headers that aim to make applications more secure. When we look at their changelog, we can see that the maintainers keep the list of headers up to date by adding new ones and deprecating headers that are n ..read more
Wanago » TypeScript
3M ago
Command Line Applications are very useful for developers. We can interact with them by executing specific commands in the terminal, giving us much control and flexibility. You probably already interact with various CLI applications, such as Git or NPM.
Interestingly, we can also use NestJS to create a Command Line Interface (CLI) application. In this article, we learn what we can do with CLI apps and how to implement one with NestJS and the Nest Commander.
Command Line Interface applications
A straightforward example of a CLI tool is cp, which is used to copy files.
cp file.txt copiedFil ..read more
Wanago » TypeScript
3M ago
Some of our SQL queries can become quite complicated. Fortunately, we can create views that act as aliases for the select queries. They have a form of virtual tables with rows and columns that we can select from but can’t insert any data into. We can also use materialized views that store the query results in the database, effectively creating a cached data version. In this article, we learn how to create and use views using PostgreSQL, NestJS, and Kysely.
If you want to know more about the basics of using NestJS with PostgreSQL and Kysely, check out API with NestJS #119. Type-safe SQL querie ..read more
Wanago » TypeScript
3M ago
Let’s say two people want to video chat using our app. One solution would be for the first person to stream their camera feed to our server. Then, our application would pass this data to the other caller. Unfortunately, our application acting as a middleman could introduce a significant lag, especially if our server is far from the call participants. Fortunately, we can use WebRTC to connect our users directly without sending all of the data through our server. This improves performance and helps us achieve a smooth experience.
Even if the video and audio are not streamed through our applicati ..read more
Wanago » TypeScript
4M ago
PostgreSQL allows us to filter a query’s results and ensure we don’t get duplicate rows. This can be helpful when your table has many rows where the data in the columns is the same. In this article, we explore two ways PostgreSQL helps us solve this problem. We also learn how to do that with Prisma and configure it to use the native database features instead of filtering the duplicates in memory.
The DISTINCT keyword
Let’s say we have the following model created with Prisma.
schema.prisma
model Address {
id Int @id @default(autoincrement())
street String
city String
countr ..read more
Wanago » TypeScript
4M ago
In PostgreSQL, schemas act as namespaces within the database and are containers for objects such as tables and indexes. In this article, we explain how they work and what are their benefits. We also provide examples of how to use them with Prisma.
The public schema
PostgreSQL creates a schema called public out of the box for every new database. Let’s say we have the following Article model.
schema.prisma
model Article {
id Int @id @default(autoincrement())
title String
content String
}
When we generate a migration, we can see that it does not mention any schemas at all.
If y ..read more
Wanago » TypeScript
4M ago
Each record in our database should have a unique identifier. Typically, we use a numerical sequence to generate them. However, we can use an alternative approach that includes Universally Unique Identifiers (UUID). In this article, we discuss their advantages and disadvantages and implement them in a project with NestJS, Prisma, and PostgreSQL.
For the full code from this article check out this repository.
The idea behind UUID
A Universally Unique Identifier is a number represented using the hexadecimal system. While we’re used to the decimal system, which uses ten symbols (0-9) for values ..read more