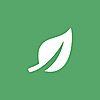
Baeldung » Spring
271 FOLLOWERS
Have a look through a list of in-depth tutorials on the various Spring modules, including Spring MVC, Spring Security, Spring Cloud and many more. Baeldung helps developers explore the Java ecosystem and simply be better engineers. We publish to-the-point guides and courses, with a strong focus on building web applications, Spring, Spring Security, and RESTful APIs.
Baeldung » Spring
1w ago
1. Overview
Interceptors, also known as filters, are a feature in Spring that allows us to intercept client requests. This allows us to examine and transform the request before the controller handles it or returns response to the client.
In this tutorial, we’ll discuss various ways of intercepting a client request and adding custom headers using the WebFlux Framework. We’ll first explore how to do it for a specific endpoint. Then, we’ll determine the approach for intercepting all incoming requests.
2. Maven Dependency
We’ll be using the following spring-boot-starter-webflux Maven dependency fo ..read more
Baeldung » Spring
2w ago
1. Introduction
In this article, we’ll explore how to retrieve the response body from a ServletResponse in a Spring Boot filter.
In essence, we’ll define the problem, and then we’ll use a solution that caches the response body to make it available in the Spring Boot filter. Let’s begin.
2. Understanding the Problem
First, let’s understand the problem we are trying to solve.
When working with Spring Boot filters, it is tricky to access the response body from the ServletResponse. This is because the response body is not readily available, as it is written to the output stream after the filt ..read more
Baeldung » Spring
2w ago
1. Overview
Error handling is a critical aspect of reactive programming with Spring WebFlux. Developers usually rely on two primary methods for error handling: throwing exceptions or using the Mono.error() method provided by Project Reactor. Both approaches are used to signal errors, but they have distinct characteristics and use cases.
In this tutorial, we will explain the differences between throwing an exception and Mono.error() in Spring WebFlux. We’ll provide illustrative Java code examples to make it more understandable.
2. Traditional Approach: Throwing Exceptions
For many years, throwi ..read more
Baeldung » Spring
2w ago
1. Introduction
Caching is an effective strategy to improve performance by avoiding repeated execution of logic when the execution result hasn’t changed (effectively the same) for a known period.
Spring Boot provides the @Cacheable annotation, which we define over a method, and it caches the result of the method. In some scenarios, such as during testing in a lower environment, we may need to disable caching to observe certain modified behaviors.
In this article, we’ll configure the cache in Spring Boot and learn how to disable the cache when needed.
2. Caching Setup
Let’s set up a simple use ..read more
Baeldung » Spring
2w ago
1. Introduction
In this tutorial, we’ll dive into the topic of testing Spring applications which utilize scheduled tasks. Their extensive usage might cause a headache when we try to develop tests, especially integration ones. We’ll talk about possible options to make sure that they’re as stable as they can be.
2. Example
Let’s start with a short explanation of the example we’ll be using throughout the article. Let’s imagine a system that allows the representative of the company to send notifications to their clients. Some of them are time-sensitive and should be delivered immediately, but some ..read more
Baeldung » Spring
1M ago
1. Introduction
Grafana Labs developed Loki, an open-source log aggregation system inspired by Prometheus. Its purpose is to store and index log data, facilitating efficient querying and analysis of logs generated by diverse applications and systems.
In this article, we’ll set up logging with Grafana Loki for a Spring Boot application. Loki will collect and aggregate the application logs, and Grafana will display them.
2. Running Loki and Grafana Services
We’ll first spin up Loki and Grafana services so that we can collect and observe logs. Docker containers will help us to more easily configu ..read more
Baeldung » Spring
1M ago
1. Introduction
In a typical microservice-based architecture, where a single business use case spans multiple microservices, each service has its own local datastore and localized transaction. When it comes to multiple transactions, and the number of microservices is vast, there comes the requirement to handle the transaction spanning various services.
The Saga Pattern was introduced to handle these multiple transactions. Initially introduced in 1987 by Hector Garcia Molina and Kenneth Salems, it’s defined as a sequence of transactions that can be interleaved with one another.
In this tutorial ..read more
Baeldung » Spring
1M ago
1. Overview
In this quick article, we’re going to learn about creating a prototype-scoped bean with runtime arguments in Spring.
In Spring, there are many different bean scopes, but the default scope is singleton, which means that singleton-scoped beans will always produce the same object.
Alternatively, we can use the prototype-scoped bean if we need a new instance from the container every time. However, in most cases, we encounter issues if we want to instantiate the prototype from a singleton bean or transfer the dynamic parameter to prototype beans.
Spring provides many methods to achieve ..read more
Baeldung » Spring
1M ago
1. Introduction
In the realm of database management, ensuring consistency and traceability of schema changes is a good practice for maintaining data integrity and application reliability. Liquibase, a widely adopted database schema change management tool, helps us version control and track database changes seamlessly across various environments.
However, as applications grow in complexity, it becomes imperative to organize database objects efficiently. While PostgreSQL defaults to the public schema, best practices advocate for segregating application-specific entities into dedicated schemas.
I ..read more
Baeldung » Spring
1M ago
1. Overview
In this tutorial, we’ll explore how to dynamically register beans based on custom properties. We’ll explore the BeanDefinitionRegistryPostProcessor interface and how we can use it to add beans to the application context.
2. Code Setup
Let’s start by creating a simple Spring Boot application.
First, we’ll define a bean that we want to register dynamically. Then, we’ll provide a property that decides how to register the beans. And finally, we’ll define a configuration class that will register the bean based on our custom properties.
2.1. Dependencies
Let’s start by adding the Ma ..read more