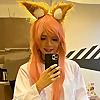
Ruby Yagi
20 FOLLOWERS
Howdy! My name is Axel Kee. I am a software developer based in Kuala Lumpur, Malaysia. I used to work on iOS using Objective-C and Swift, now my day job is as a developer (mainly Ruby/Rails, frontend and Ansible) for an enterprise. I hope to share my web development experience on this blog, and hope it can help future readers.
Ruby Yagi
2y ago
Ruby has some useful methods for enumerable (Array, Hash, etc), this post will talk about usage of any?, all?, and none?.
For examples used on this post, an Order model might have many Payment model (customer can pay order using split payments).
any?
To check if an order has any paid payments, a loop-based implementation might look like this :
has_paid_payment = false
order.payments.each do |payment|
if payment.status == "paid"
# one of the payment is paid
has_paid_payment = true
break
end
end
if has_paid_payment
# ...
end
We can simplify the code above using any? like t ..read more
Ruby Yagi
2y ago
If you are working on an app that has user generated content (like blog posts, comments etc), there might be scenario where it is too long to display and it would make sense to truncate them and put a “read more” button below it.
This post will solely focus on the front end, which the web browser will detect if the text needs to be truncated, and only show read more / read less as needed. (dont show “read more” if the text is short enough)
TL;DR? Check out the Demo at CodePen.
Truncate text using CSS
Referenced from TailwindCSS/line-clamp plugin.
Using the combination of these CSS properties ..read more
Ruby Yagi
2y ago
Here’s a method I encountered recently at my work and I found it might be useful to you as well.
There might be some attribute which you want to set to a default parameter if the user didn’t supply any, eg:
# if the parameters is nil or blank, we will default to french fries
sides = params[:sides].present? ? params[:sides] : "french fries"
This one liner looks concise, but we can make it even shorter like this :
sides = params[:sides].presence || "french fries"
The presence method will return nil if the string is a blank string / empty array or nil , then the || (double pipe) will use th ..read more
Ruby Yagi
2y ago
How to get started with TailwindCSS
This article assumes you have heard of TailwindCSS, and interested to try it out but have no idea where to start.
Try it out online on Tailwind Play
If you just want to try out TailwindCSS and don’t want to install anything on your computer yet, the official Tailwind Play online playground is a good place to start!
Try it on your HTML file without fiddling with the nodeJS stuff
This section is for when you want to use TailwindCSS on a static website, but you don’t want to deal with the nodeJS NPM stuff.
Thankfully, Beyond Code has released TailwindCSS JIT vi ..read more
Ruby Yagi
2y ago
If you have been writing Ruby / Rails code for a while, but still can’t grasp on the ‘why’ to write automated test, (eg. why do so many Ruby dev job posting requires automated test skill like Rspec, minitest etc? How does writing automated test makes me a better developer or help the company I work with? )
or if you are not sure on how the CI (continuous integration) stuff works, this article is written to address these questions!
This article won’t go into how to write unit test, but it will let you experience what is it like working on a codebase that has automated testing in place, and how ..read more
Ruby Yagi
2y ago
I was working on a feature for my Rails app, which allows merchant to set fulfillment times on each day like this :
The main feature is that once an order is placed, the customer can’t cancel the placed order after the fulfillment time starts on that day (or on tomorrow).
The fulfillment start times are saved in different columns like this :
create_table "fulfillments", force: :cascade do |t|
t.time "monday_start_time", default: "2000-01-01 00:00:00", null: false
t.time "tuesday_start_time", default: "2000-01-01 00:00:00", null: false
t.time "wednesday_start_time", default: "2000-01-0 ..read more
Ruby Yagi
2y ago
How to run tests in parallel in Github Actions
The company I work for has recently switched to Github Actions for CI, as they offer 2000 minutes per month which is quite adequate for our volume. We have been running tests in parallel (2 instances) on the previous CI to save time, and we wanted to do the same on Github Actions.
Github Actions provides strategy matrix which lets you run tests in parallel, with different configuration for each matrix.
For example with a matrix strategy below :
jobs:
test:
name: Test
runs-on: ubuntu-latest
strategy:
matrix:
ruby ..read more
Ruby Yagi
2y ago
Sometimes for security purpose, or you just dont want users to share their accounts, it is useful to implement a check to ensure that only one login (session) is allowed per user at the same time.
To check if there is only one login, we will need a column to store information of the current login information. We can use a string column to store a token, and this token will be randomly generated each time the user is logged in, and then we compare if the current session’s login token is equal to this token.
Assuming your users info are stored in the users table (you can change to other name for ..read more
Ruby Yagi
2y ago
TailwindCSS v2.0 was released on 19 November 2020 ?, but it had a few changes that requires some tweak to install and use it on Rails 6, such as the requirement of PostCSS 8 and Webpack 5. As of current (10 December 2020), Rails 6 comes with webpack 4 and PostCSS 7, which doesn’t match the requirement of TailwindCSS v2.
If we install TailwindCSS v2 directly on a Rails 6 app using yarn add tailwindcss , we might get a error (PostCSS plugin tailwindcss requires PostCSS 8) like this when running the server :
This tutorial will guide you on how to install and use TailwindCSS 2 on Rails 6 using n ..read more
Ruby Yagi
2y ago
Most of the time when we implement API endpoints on our Rails app, we want to limit access of these API to authorized users only, there’s a few strategy for authenticating user through API, ranging from a simple token authentication to a fullblown OAuth provider with JWT.
In this tutorial, we will implement an OAuth provider for API authentication on the same Rails app we serve the user, using Devise and Doorkeeper gem.
After this tutorial, you would be able to implement Devise sign in/sign up on Rails frontend, and Doorkeeper OAuth (login, register) on the API side for mobile app client, or a ..read more