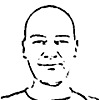
C++ Stories
228 FOLLOWERS
Stay up-to-date with Modern C++. My name is Bartlomiej Filipek. I am a software developer from the beautiful city Cracow in Southern Poland. This is my technical blog.
C++ Stories
3d ago
std::expected from C++23 not only serves as an error-handling mechanism but also introduces functional programming paradigms into the language. In this blog post, we’ll have a look at functional/monadic extensions of std::expected, which allow us to chain operations elegantly, handling errors at the same time. The techniques are very similar to std::optional extensions - see How to Use Monadic Operations for `std::optional` in C++23 - C++ Stories.
Here’s a brief overview of these functional capabilities:
Update: Thanks to prof. Boguslaw Cyganek for finding some errors in my initial text!
and_t ..read more
C++ Stories
1w ago
In the article about std::expected, I introduced the type and showed some basic examples, and in this text, you’ll learn how it is implemented.
A simple idea with struct
In short, std::expected should contain two data members: the actual expected value and the unexpected error object. So, in theory, we could use a simple structure:
template <class _Ty, class _Err>
struct expected {
/*... lots of code ... */
_Ty _Value;
_Err _Unexpected;
};
However, there are better solutions than this. Here are some obvious issues for our “struct” approach.
The size of the object i ..read more
C++ Stories
3M ago
In this article, we’ll go through a new vocabulary type introduced in C++23. std::expected is a type specifically designed to return results from a function, along with the extra error information.
Motivation
Imagine you’re expecting a certain result from a function, but oops… things don’t always go as planned.
/*RESULT*/ findRecord(Database& db, int recordId) {
auto record = db.query(recordId);
if (record.isValid()) {
return record;
return /*??*/
}
What can you do? What should you return or output through /*RESULT*/ ?
Here are a few options:
Throw an except ..read more
C++ Stories
3M ago
Thanks to the powerful constexpr keyword and many enhancements in recent C++ standards, we can now perform a lot of computations at compile time. In this text, we’ll explore several techniques for parsing integers, including the “naive” approach, C++23, from_chars, std::optional, std::expected, and even some upcoming features in C++26.
But first… why would this even be needed?
Why at compile time?
While it may sound like a theoretical experiment, since C++11 we can shift more and more computations to compile-time. Here are some key areas and examples where constexpr can be beneficial ..read more
C++ Stories
5M ago
In this article, we’ll explore six practical string processing operations introduced in C++20 and C++23. These features represent an evolution in this crucial area, covering a spectrum of operations from searching and appending to creation and stream handling.
Let’s start with a simple yet long-awaited feature…
1. contains(), C++23
Finally, after decades of standardization, we have a super easy way to check if there’s one string inside the other. No need to use .find(str) != npos!
Before C++23:
#include <string>
#include <iostream>
int main() {
const std::string url ..read more
C++ Stories
7M ago
In this article, we’ll look at std::span which is more generic than string_view and can help work with arbitrary contiguous collections.
A Motivating Example
Here’s an example that illustrates the primary use case for std::span:
In traditional C (or low-level C++), you’d pass an array to a function using a pointer and a size like this:
void process_array(int* arr, std::size_t size) {
for(std::size_t i = 0; i < size; ++i) {
// do something with arr[i]
}
}
std::span simplifies the above code:
void process_array(std::span<int> arr_span) {
for(auto& elem : arr_sp ..read more
C++ Stories
7M ago
In this blog post, we’ll look at several different view/reference types introduced in Modern C++. The first one is string_view added in C++17. C++20 brought std::span and ranges views. The last addition is std::mdspan from C++23.
Let’s start.
String View (C++17)
The std::string_view type is a non-owning reference to a string. It provides an object-oriented way to represent strings and substrings without the overhead of copying or allocation that comes with std::string. std::string_view is especially handy in scenarios where temporary views are necessary, significantly improving the perf ..read more
C++ Stories
8M ago
Learn how the overload pattern works for std::variant visitation and how it changed with C++20 and C++23.
While I was doing research for my book and blog posts about C++17 several times, I stumbled upon this pattern for visitation of std::variant:
template<class... Ts> struct overload : Ts... { using Ts::operator()...; };
template<class... Ts> overload(Ts...) -> overload<Ts...>;
With the above pattern, you can provide separate lambdas “in-place” for visitation.
It’s just two lines of compact C++ code but packs some exciting techniques.
Let’s see how this works and go th ..read more
C++ Stories
9M ago
Today, I’ll show you my review of a cool book, “Beautiful C++,” written by two well-known C++ experts and educators: Kate Gregory and Guy Davidson. The book’s unique style gives us a valuable perspective on effective and safe C++ code.
Let’s see what’s inside.
Disclaimer: I got a free copy from the publisher.
Disclaimer 2: The links to Amazon are affiliate links, which provide me with some small commission.
The book
Here are the main links and info about the book:
The book on Amazon:
Beautiful C++: 30 Core Guidelines for Writing Clean, Safe, and Fast Code @Amazon (released December 16 ..read more
C++ Stories
9M ago
In this post we’ll have a look at new operations added to std::optional in C++23. These operations, inspired by functional programming concepts, offer a more concise and expressive way to work with optional values, reducing boilerplate and improving code readability.
Let’s meet and_then(), transform() and or_else(), new member functions.
Traditional Approach with if/else and optional C++20
In C++20 when you work with std::optional you have to rely heavily on conditional checks to ensure safe access to the contained values. This often led to nested if/else code blocks, which could make t ..read more