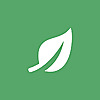
Baeldung » Java
1,269 FOLLOWERS
Baeldung helps developers explore the Java ecosystem and simply be better engineers. Java is the popular programming language that originated in 1995. Today, the Java ecosystem includes a very large number of specifications, libraries, frameworks, tools, as well as a thriving community. Take a dive through our collection of Java tutorials that cover a wide array of Java-related topics.
Baeldung » Java
2d ago
1. Overview
In this tutorial, we’ll learn how to convert a Queue object into a List in Java.
We’ll explain several popular ways of doing this along with their implementations, and we’ll end each section with a test case to test the respective implementation.
2. Ways to Convert Queue to List
In this section, we’ll cover different ways to convert the Queue to a List using standard Java classes and methods. We’re assuming a non-null queue for all examples.
2.1. Using the ArrayList Constructor
The ArrayList constructor provides the easiest and most common way to convert a Queue to a ..read more
Baeldung » Java
3d ago
1. Overview
In this tutorial, we’ll explore different methods of converting Jackson’s raw data type JsonNode into typed Java collections. While we can read JSON using JsonNode itself, converting it to a Java collection can be beneficial. Java collections provide advantages over raw JSON data such as type safety, faster processing, and availability of more type-specific operations.
2. Example Setup
In our code example, we’ll look at different ways to convert a JsonNode to a List or Map of objects. Let’s set up the building blocks of our exa ..read more
Baeldung » Java
3d ago
1. Introduction
In Java programming, dealing with collections is a fundamental task. Lists and Maps are two commonly used collection types, and sometimes, we might need to work with a List of Maps. Whether we’re processing data, manipulating configurations, or any other task that involves complex data structures, efficiently iterating through a List of Maps is crucial.
In this tutorial, we’ll explore various techniques for iterating through a List of Maps in Java.
2. Understanding List of Maps
Before we explore iteration techniques, let’s grasp the concept of a List of Maps.
A List of Maps com ..read more
Baeldung » Java
3d ago
1. Introduction
Java introduced the Optional class in Java 8 to represent a value that may or may not be present. It helps us to avoid NullPointerException and write more expressive and readable code. Converting an Optional to an ArrayList can be useful in scenarios where we want to handle optional values as lists. In this tutorial, we’ll explore different approaches to convert an Optional to an ArrayList in Java.
2. Using isPresent()
This approach leverages the isPresent() method provided by the Optional class to handle a value’s presence or absence. It’s particularly useful when we want to e ..read more
Baeldung » Java
3d ago
1. Overview
Optimizing code for performance is a key part of programming, especially when dealing with expensive operations or data retrieval processes. One effective way of improving performance is caching.
The Project Reactor library provides a cache() method to cache expensive operations or data that hardly changes to avoid repeat operations and improve performance.
In this tutorial, we’ll explore memoization, a form of caching, and demonstrate how to use Mono.cache() from the Project Reactor library to cache the results of the HTTP GET request to JSONPlaceholder API. Also, we’ll ..read more
Baeldung » Java
3d ago
1. Introduction
The need for fast and efficient text searches often arises when developing database applications. They should also support full and partial text matches to make these searches even more user-friendly. For this purpose, MongoDB provides a couple of approaches to finding relevant documents using text searches.
In this tutorial, we’ll explore text search in MongoDB, its features, how to use it, and how to get the most out of it.
2. Text Search in MongoDB
While text search queries are a powerful tool, they require a specific setup. To achieve this, we’ll need to create a text index ..read more
Baeldung » Java
4d ago
1. Introduction
Inheritance and composition are two fundamental concepts in object-oriented programming (OOP) that we can also leverage in JPA for data modeling. In JPA, both inheritance and composition are techniques for modeling relationships between entities, but they represent different kinds of relationships. In this tutorial, we’ll explore both approaches and their implications.
2. Inheritance in JPA
Inheritance represents an “is-a” relationship, where a subclass inherits properties and behaviors from a superclass. This promotes code reuse by allowing subclasses to inherit attributes and ..read more
Baeldung » Java
4d ago
1. Introduction
Converting a short value to a byte[] array is a common task in Java programming, especially when dealing with binary data or network communication.
In this tutorial, we’ll explore various approaches to achieve this conversion efficiently.
2. Using ByteBuffer Class (Java NIO)
The Java NIO package provides the ByteBuffer class, which simplifies the conversion of primitive data types into byte arrays. Let’s take a look at how we can use it to convert a short value to a byte[] array:
short shortValue = 12345;
byte[] expectedByteArray = {48, 57};
@Test
public void givenShort_whenUs ..read more
Baeldung » Java
4d ago
1. Introduction
In this tutorial, we’ll continue to explore OpenAPI Generator‘s customization options. This time, we’ll show how the required steps to create a new generator that creates REST Producer routes for Apache Camel-based applications.
2. Why Create a New Generator?
In a previous tutorial, we’ve shown how to customize an existing generator’s template to suit a particular use case.
Sometimes, however, we’ll be faced with a situation where can’t use any of the existing generators. For example, this is the case when we need to target a new language or REST framework.
As a concrete exampl ..read more
Baeldung » Java
4d ago
1. Overview
Sometimes we deal with entities with complex models consisting of parent and child elements. In this scenario, it can be beneficial to save the parent entity, thereby automatically saving all its children entities.
In this tutorial, we’ll delve into various aspects that we might otherwise overlook in achieving this automatic saving. We’ll discuss both unidirectional and bidirectional relationships.
2. Missed Relationships Annotations
The first thing we might overlook is adding a relationships annotation. Let’s create a child entity:
@Entity
public class BidirectionalChild {
@I ..read more