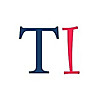
Tech Incent
591 FOLLOWERS
Hi! I'm Md. Sajal Mia. I am a highly skilled and experienced web developer with a passion for creating visually appealing and user-friendly websites. With several years of experience in the field, I have a strong understanding of web development technologies and methodologies and am able to deliver high-quality, performant, and responsive websites that meet the needs of my clients. Tech..
Tech Incent
5M ago
Insertion Sort is a simple sorting algorithm that builds the final sorted array one item at a time. It iterates through the given array and, at each iteration, it takes an element and inserts it into the correct position in the already sorted part of the array.
Here’s how the Insertion Sort algorithm works:
Start from the second element (index 1) of the array since the first element (index 0) is considered initially as a sorted part of the array.
Iterate through the array: For each element in the unsorted part of the array, compare it with the elements in the sorted part of the array, moving ..read more
Tech Incent
5M ago
Selection sort is a simple sorting algorithm that repeatedly selects the smallest (or largest, depending on sorting order) element from an unsorted portion of the list and swaps it with the element in the next position of the sorted portion of the list. This process continues until the entire list is sorted.
Explanation of how selection sort works:
Selection of the Smallest Element: The algorithm divides the input list into two parts: the sorted part at the beginning and the unsorted part at the end. Initially, the sorted part is empty, and the unsorted part contains the entire list.
Find th ..read more
Tech Incent
5M ago
Bubble sort is a simple comparison-based sorting algorithm that repeatedly steps through the list, compares adjacent elements, and swaps them if they are in the wrong order. This process is repeated until the entire list is sorted.
How the bubble sort algorithm works:
Iterate Through the List:
Start at the beginning of the list.
Compare the first two elements. If they’re in the wrong order, swap them.
Repeat Comparison and Swapping:
Move to the next pair of elements and compare them.
Continue this process, moving through the list, comparing adjacent elements, and swapping them if necessar ..read more
Tech Incent
5M ago
Circular queues are a fundamental data structure that follows the First-In-First-Out (FIFO) principle, but with a unique twist—the last element is connected to the first, forming a circular structure. In this article, we’ll delve into the TypeScript implementation of a circular queue, exploring its key components and understanding how it efficiently manages data.
Understanding Circular Queues
A circular queue consists of a fixed-size array and two pointers: a front pointer (head) and a rear pointer (tail). The front represents the beginning of the queue, and the rear represents the end. Unlike ..read more
Tech Incent
6M ago
In this article, I will implement pagination and search for large amounts of data. This pagination and search will be optimized with the debounce technique. I also use some of RxJs operators like debounceTime, distinctUntilChanged, switchMap, exhaustMapcand more. And I will use fake product pagination data API from StoreRestApi.
Explanation search feature
Our goal for the search is, there will be a search field that takes input value. When the user inputs some value after .5s from stop input values that will call API.
We must be aware of every input change API calling, For example, if the sear ..read more
Tech Incent
6M ago
If you’re looking to implement memorization in JavaScript, you’re likely referring to memoization, which is a technique used to optimize functions by caching their results based on their input parameters. This can help avoid unnecessary computations and improve the performance of your code
// Example of higher order function
// Memorization
/*
Callback function for reduce
*/
function reduceCallback(sum, element) {
return sum + element
}
const total = function(...circle) {
const _total = circle.reduce(reduceCallback)
return _total;
};
// console.log(total(...radius))
// Higher Orde ..read more
Tech Incent
9M ago
A higher-order function in JavaScript is a function that can take one or more functions as arguments and/or return a function as its result. Essentially, it treats functions as first-class citizens, allowing them to be manipulated and passed around just like any other data type.
Here’s an example to illustrate the concept:
// Example of a higher-order function
function higherOrderFunction(func) {
console.log("Executing the higher-order function");
func(); // Calling the function passed as an argument
}
// Function to be passed as an argument
function myFunction() {
console.log("Hello f ..read more
Tech Incent
10M ago
In this article, I will implement an angular router resolver with an explanation. So we will create two component product list and production description. Product List will have a product list resolver and the other will be a product description resolver which takes the product slug from the current route param.
So what is an angular resolver?
Angular resolver is an injectable service class that gives pre-data before component init. Resolver used for pre-fetching data after angular route end.
So how is an angular resolver implemented?
An angular resolver implements by Implementing an angular R ..read more
Tech Incent
10M ago
Using the binary search takes less time than a linear search. In this article, I explain binary search with typescript based on my learning
What is the working principle of the binary search?
One prerequisite, or one requirement for the binary search, is that the Array of data must be sorted. If array data is not sorted, you can’t apply binary search. So you need to sort data first then you can apply
An essential point of binary search is Divide And Conquer Technique, What are the means? It’s going to divide the array into two halves recursively. Imagine the scenario we have a sorted array and ..read more
Tech Incent
10M ago
In this guide, we’ll concentrate on the following abilities:
Using Bootstrap’s style and feel to build UI in a React-based web app.
Creating a React app for listing contacts with reactstrap.
The most popular JS framework for creating dynamic web apps is React. However, because it is a view library, it does not provide approaches for creating responsive and intuitive designs. We may use Bootstrap, a front-end design framework to solve this problem.
What is Bootstrap in React?
React-bootstrap is a library for creating front-end stylesheets. This library is made up of two libraries, one of whic ..read more