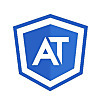
Angular Training Blog
300 FOLLOWERS
Learn about the Angular framework with tutorials, guides, tips and tricks on Angular Training Blog.
Angular Training Blog
2d ago
RxJs is the most challenging library of the Angular ecosystem because of its syntax, numerous operators, and the asynchronous mindset associated with Observables.
Today, let’s see the easiest way to debug RxJs code in Angular. Now, you’ve probably done something like this at least once:
service.getSomeObservable().subscribe(data => { console.log(data);});
While the above works, it’s not ideal for a bunch of reasons:
It forces you to subscribe to an Observable instead of using the async pipe, which is much better.
It doesn’t allow you to debug what happens in that Observa ..read more
Angular Training Blog
1M ago
Have you ever tried examining the size of an entire Angular project and comparing it to the size of the code compiled by Angular (its build output in the dist folder)?
The difference is almost hard to believe. Typically, the entire project folder of an Angular app is well above 600MB, which includes a lot of things, including your code, all your dependencies, but also the Angular CLI, the TypeScript compiler, and more:
After running ng build, your dist folder is much smaller than 600MB, usually just a few KB or MB, depending on the size of your application.
This is because of a tec ..read more
Angular Training Blog
2M ago
I've heard this question several times over the past few weeks, so I'm writing a blog post about it. At first, I saw this question as similar to asking someone, "What is your favorite color?" because I believe that every single person/dev team has their own opinions and preferences.
That said, I realized people ask that question because of possible misconceptions about how Angular works, making this topic much more interesting. Also, we're lucky enough to have an official Angular style guide to help us make informed decisions about it.
Here is my take on that topic in a Q&A form ..read more
Angular Training Blog
3M ago
In a past post, I discussed the importance of signal-based components and how such components will change the way we architect Angular applications for better performance.
I’ve also mentioned the computed() function used to derive values from any number of signals. For instance:
name = signal("Al");lastName = signal("Test");fullName = computed(() => this.name() + " " + this.lastName());
In the above example, changing the value of either name or lastName will automatically update the value of fullName.
What happens with computed() is that any signal read inside the computed function becom ..read more
Angular Training Blog
4M ago
With the release of Angular 17.3, signal-based components have become a reality. A signal-based component is one in which all data inputs, outputs, and queries are independent of RxJs and use Angular Signals instead.
In other words, here is how we used to write Angular components:
import { AsyncPipe } from '@angular/common';import { Component, EventEmitter, Input, Output, ViewChild } from '@angular/core';@Component({//...})export class HelloComponent { @Input() name = 'World'; @Output() greetingClicked = new EventEmitter<string>(); @ViewChild(ProfileComponent) profileCompone ..read more
Angular Training Blog
5M ago
A forest of cypress trees, Cyberpunk style — generated with Nightcafe
Cypress is an excellent option for end-to-end testing, and you can learn more about the basics of Cypress with this tutorial.
In this post, I will cover how to test individual components with Cypress, an available option for Angular, React, Svelte, and Vue.js.
What’s the difference between end-to-end testing and component testing?
While Cypress commands and features remain the same, these are two distinct testing modes within Cypress:
End-to-end testing loads the entire web application in a browser. Component test ..read more
Angular Training Blog
6M ago
With the release of Angular Signals, RxJS-based state management libraries had to adapt and create signal-based alternatives, which NgRx has done with its version 17.
In this post, we’re diving into how to use NgRx SignalStore, the syntax and the different options, and how different it is from regular signals.
My example comes from my free Angular Signals workshop, which is now available on YouTube.
How to install NgRx SignalStore?
This is the easiest step. Run that command in your Angular project:
npm install @ngrx/signals
Note that NgRx SignalStore is independent of Ng ..read more
Angular Training Blog
7M ago
Angular logo streaming server-side code to a browser — an AI-generated image edited by yours truly
In this post, I cover how to do server-side rendering (SSR) with Angular, why and when it matters, and everything you need to know to get started.
Why server-side rendering (SSR)?
There are a few good reasons to use SSR:
Make your application faster: If your app has a lot of static content that is always the same (not user-specific), it makes sense to have that content built on the server side and returned to all users because the server can (and will) cache that content, which pr ..read more
Angular Training Blog
8M ago
Angular is all about components, but we need to remember that directives can be a better option occasionally.
To illustrate this, here is an example of a feature we want to implement:
Detect when the user clicks outside of an element/component
Send an event to the parent component when that happens
This feature can be helpful if you want to close a dropdown, a dialog, or a date picker when the user clicks away from it.
First, we generate a directive and inject a reference to its native HTML element:
@Directive({ selector: '[appClickOutside]', standalone: true})export class ..read more
Angular Training Blog
9M ago
Angular 17 has an updated logo and brand identity, a new website (angular.dev), and many more features that will be covered in my Daily Angular Newsletter over the next few days.
In this article, let’s cover the new control flows available in our Angular HTML templates.
New “If — then — else” syntax
We’re used to the ngIf directive and its cumbersome “else” syntax as follows:
<div *ngIf="condition; else elseBlock"> Content to render when the condition is true.</div><ng-template #elseBlock> Content to render when the condition is false.</ng-template> ..read more