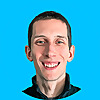
Code With Andrea
373 FOLLOWERS
Learn how to become a Flutter Pro and build production-ready apps on mobile and beyond. Hello, I'm Andrea and I'm a Google Developer Expert for Dart & Flutter. I created my website to help you become a Flutter Pro and make high-quality apps.
Code With Andrea
2d ago
How do you create a Flutter card layout that grows horizontally up to a given width and then remains fixed at that width, like in this example?
This can be done by composing Center and SizedBox widgets like so:
class ResponsiveCenter extends StatelessWidget { const ResponsiveCenter({super.key, this.maxWidth = 600, required this.child}); final double maxWidth; final Widget child; @override Widget build(BuildContext context) => Center( child: SizedBox( width: maxWidth, child: child, ), ); }
Then, to recreate the card layout from above, you can use this code:
class CenteredCardLayout extend ..read more
Code With Andrea
5d ago
Ever wanted to filter tests so you only run the ones you need to?
This can be easily done using test tags.
Super useful when you have dozens/hundreds of tests and you want to target specific ones from the command line. ?
Example Code
import 'package:flutter_test/flutter_test.dart'; void main() { testWidgets( 'Golden image test', (tester) async { await tester.pumpWidget(const MyApp()); await expectLater( find.byType(MyApp), matchesGoldenFile('golden_image.png'), ); }, tags: ['golden'], ); }
Configuration Options
Tags are quite flexible and you can use them to tag:
an entire test suite (with th ..read more
Code With Andrea
5d ago
The Dart ecosystem equips developers with a variety of powerful tools that provide a blazingly fast and productive development experience. Yet, there is an opportunity to push these capabilities further by adopting techniques like code generation.
This guide will cover all aspects of integrating code generation in day-to-day development, including:
Code generation mechanism: we’ll discuss how the code generation mechanism with build_runner works.
Useful code-generating packages: we’ll look into useful packages such as json_serializable, freezed, retrofit, injectable, bdd_widget_test, barrel_f ..read more
Code With Andrea
5d ago
Did you know?
You can run a widget test multiple times with different variants using the variant argument.
This is quite useful if you have a responsive app and want to run a golden image test for different screen sizes. ?
Example Code
import 'dart:ui'; import 'package:flutter_test/flutter_test.dart'; void main() { final sizeVariant = ValueVariant<Size>({ const Size(300, 600), const Size(600, 800), const Size(1000, 1000), }); testWidgets( 'Golden - products list', (tester) async { final r = Robot(tester); final currentSize = sizeVariant.currentValue!; await r.golden.setSurfaceSize(curren ..read more
Code With Andrea
1w ago
When writing test expectations for functions that throw, we need a bit careful.
If we call the function directly, the test will fail as it won't catch the exception as we expect.
In these cases, we should always pass a closure when calling the expect method as shown here. ?
Here's a comparison I made to explain why calling the method directly won't work.
These two implementations are equivalent. And they both fail because the function will execute and throw before expect() is even called. ?
Hope this will save you some headaches ..read more
Code With Andrea
1w ago
Only a few weeks are left until Google I/O, kicking off on May 14th.
I bet the main focus will be on Google’s latest AI advancements. But we can also expect a new Flutter release, and I hope to hear some updates on WASM support and Dart Macros.
But for now, let’s focus on this newsletter and the latest videos, articles, packages, and tools from the Flutterverse.
Flutter Videos
This month, I have three new videos for you.
? Building a Flutter Calculator: Part 1, The Layout
Back in my January newsletter, I shared a video by indie dev Andrei Lupsa, who made a calculator app with 10M downloads on ..read more
Code With Andrea
1w ago
Flutter tip: use composition aggressively
Most widgets in the Flutter SDK use composition aggressively.
By doing the same in our own apps, we can create small, reusable widgets that are easier to reason about.
Embrace this and banish the massive build method from existence! ?
Your future self will thank you. ?
Column( crossAxisAlignment: CrossAxisAlignment.stretch, children: [ Text(product.title, style: Theme.of(context).textTheme.titleLarge), gapH8, Text(product.description), // Only show average if there is at least one rating if (product.numRatings >= 1) ...[ gapH8, ProductAverageRating ..read more
Code With Andrea
1w ago
One of the most best things you can do for your Flutter project is to run all the tests when you push your code.
And with GitHub workflows, this can be accomplished in 10 lines of code.
Just create a tests.yaml file and put it in .github/workflows.
There is SO MUCH more that you can do with GitHub workflows!
This page covers the entire workflows syntax. 100% worth it:
Workflow syntax for GitHub Actions
Adding a tests workflow to your repo
To add a basic test worflow to your project, create a .github/workflows/tests.yaml file with these contents:
name: Run Tests on: [push, workflow_dispatch ..read more
Code With Andrea
1w ago
Did you know?
You can easily add gaps inside Flex widgets such as Columns and Rows or scrolling views.
Just install the gap package, and replace all those pesky SizedBoxes!
When to Choose Gap vs SizedBox
Note that Gap(x) is a valid substitute for both SizedBox(width: x) and SizedBox(height: x), because it works along the main axis of the parent widget, and its syntax is shorter.
But of course, it's a 3rd-party package that adds an extra dependency to your project.
If you feel it's not justified, don't use it. Again, SizedBox is just fine.
Constant Gap Widgets with SizedBox
In my apps, I prefer ..read more
Code With Andrea
3w ago
In mobile app development, fetching data efficiently is crucial for a smooth user experience. Imagine your app has to display a list of items fetched from an API. If the list is massive, retrieving all items at once could cause significant problems, including slow load times, high memory usage, and excessive data consumption. This is where pagination comes to the rescue.
Pagination divides large datasets into manageable chunks or pages, allowing the app to load a specific subset of data at a time. This approach reduces the initial payload and improves the user experience by offering a more res ..read more