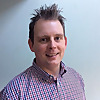
Carl Rippon Blog
112 FOLLOWERS
My name is Carl Rippon, I've been working in the software industry for over 20 years now, developing lines of business applications in various sectors. I build products using React, TypeScript, and ASP.NET Core and share useful content about these technologies as I solve problems and learn new features that are released.
Carl Rippon Blog
1y ago
Dark mode is a feature that allows users to choose a dark color scheme and is supported in macOS, iOS, Android, and Windows 10. In this post, we will cover how dark mode support can be added to a React app with the help of CSS properties.
Getting the preferred color scheme from the OS
The users preferred colour scheme from the OS is held within the prefers-color-scheme CSS media feature. We can get the value of this using a matchMedia method on the Window object in JavaScript:
const darkOS = window.matchMedia(
"(prefers-color-scheme: dark)"
).matches;
To test this in Chrome, we can set p ..read more
Carl Rippon Blog
1y ago
In this post, we will test an ASP.NET Core Web API controller with a SQL backend using xUnit. All the tests will exercise the SQL database rather than mock it out. This will quickly give a good amount of coverage and confidence that all the Web API layers are working together correctly.
Controller to test
We are going to implement some tests on the following action methods within the controller.
public async Task<IEnumerable<Product>> GetAll()
{
...
}
public async Task<ActionResult<Product>> GetById(Guid productId)
{
...
}
public async Task<ActionResult ..read more
Carl Rippon Blog
1y ago
Testing apps on accessibility is something that is often forgotten. This post covers a couple of tools that provide automated accessibility checks for React apps.
eslint-plugin-jsx-a11y
The first tool is an ESLint plugin called eslint-plugin-jsx-a11y.
To install the accessibility plugin, run the following command in a terminal:
npm install eslint-plugin-jsx-a11y --save-dev
We tell ESLint to use the accessibility plugin with its recommended rules in the ESLint configuration file as follows:
{
...,
"plugins": [..., "jsx-a11y"],
"extends": [..., "plugin:jsx-a11y/recommended ..read more
Carl Rippon Blog
1y ago
Redux is a popular library used to manage state in React apps. In this post, we’ll use Redux to manage some state in an app in a strongly-typed fashion with TypeScript. We’ll use the hooks approach to interact with the Redux store from a React component.
State
Let’s start with the stores state object:
type Person = {
id: number;
name: string;
};
type AppState = {
people: Person[];
};
So, our app state contains an array of people.
This app is going to be deliberately simple so that we can focus on the Redux Store and how a React component interacts with it in a strongly-typed manner ..read more
Carl Rippon Blog
1y ago
When developing a reusable component, there are cases when we want to warn consumers when they pass a bad props combination. This post covers how to mock console.warn in a test and verify a warning appears for a bad props combination.
A component to test
The following component warns consumers when the value and defaultValue props are both passed:
export function Input(props: React.ComponentPropsWithoutRef<"input">) {
React.useEffect(() => {
if (props.value !== undefined && props.defaultValue !== undefined) {
console.warn(
"If you are controlling `value ..read more
Carl Rippon Blog
1y ago
Emotion is a popular CSS-in-JS library for React. One of the things I love about it is the ability to declare conditional styles directly on the component using the css prop.
Conditional styles contain logic that we may want to cover in our component tests. This post covers how to do this.
A component with conditional styles
Here’s a Button component with a conditional background colour. The condition is dependent on whether it is a primary button or not:
type Props = React.ComponentPropsWithoutRef<"button"> & {
primary?: boolean;
};
export function Button({ primary, ...rest ..read more
Carl Rippon Blog
1y ago
This post will cover what the JavaScript stopPropagation and stopImmediatePropagation methods are in event parameters and the difference between them. We will also cover what event capturing is and how it impacts these methods.
stopPropagation
A recent post covered event bubbling. This is the process of executing an event handler on an element, and then its parent and then its parent’s parent …
The stopPropagation method is available in an event parameter object. When called in an event handler, it stops the bubbling process.
For example, if we have the following HTML:
<div class="contai ..read more
Carl Rippon Blog
1y ago
An example
There are cases when we want to pass additional data to a React event handler. Consider the example below:
const List = ({
items,
}: {
items: string[],
}) => {
const handleClick = () => {
console.log(
"How do I get the item related to the button?"
);
};
return (
<ul>
{items.map((item) => (
<li key={item}>
<span>{item}</span>
<button onClick={handleClick}>
Log
</button>
</li>
))}
</ul>
);
};
We want to pass the list item d ..read more
Carl Rippon Blog
1y ago
Sometimes we need to do things imperatively in React - for example, setting focus to an input element.
A useRef hook allows us to access HTML elements and invoke their methods imperatively. There is also forwardRef for forwarding the ref from a reusable component.
What if we want to use the ref internally within a reusable component and also forward the ref? This post covers how to do this.
A reusable Input component
We have a reusable Input component that contains an input element with a tooltip:
type Props = React.ComponentPropsWithoutRef<"input"> & {
tooltip?: React.ReactNode ..read more
Carl Rippon Blog
1y ago
A codemod is an automated way of making modifications to code. I think of it as find and replace on steroids.
Codemods are helpful for library maintainers because they allow them to deprecate and make breaking changes to the public part of the API while minimising upgrade costs for developers consuming the library.
For example, Material UI has the following codemod for updating to version 5.
npx @mui/codemod v5.0.0/preset-safe <path>
This post covers creating codemods for a reusable React and TypeScript component library.
Photo by Michael Aleo on Unsplash
jscodeshift
jscodeshift is a ..read more