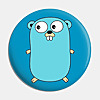
Golang By Example
625 FOLLOWERS
Blog posts cover Golang basics, Golang advanced topics, design patterns, and Data structures in Golang with examples.
Golang By Example
5M ago
groups command on linux can be used to know which all groups a user belongs to
Just type in groups on the terminal. Below is the output on my Mac machine
staff everyone localaccounts _appserverusr admin
The post Check all groups that current logged in user belong to on Linux appeared first on Welcome To Golang By Example ..read more
Golang By Example
9M ago
Overview
In the Ruby language, strings “true” and “false” are interpreted as true when used in the if condition. See example below
if "false"
puts "yes"
end
Output
"yes"
“false” evaluates to true
Therefore it becomes important to handle string “false” or string “true” correctly.
We can create a custom method that can return boolean true or false based on the content of the string
def true?(str)
str.to_s.downcase == "true"
end
We can try out the above function
true?("false")
=> false
true?("true")
=> true
Also, note that for strings other than “false” it will give true in ..read more
Golang By Example
9M ago
Overview
Linux command ‘whoami’ can be used to know the currently logged-in user.
Let’s see this command in action. Go to the terminal and type in the command
whoami
It will print the current user. Let’s say the current user is john then running the above command will simply print john
Output
whoami
john
The post Know the current user on Linux appeared first on Welcome To Golang By Example ..read more
Golang By Example
1y ago
Overview
In this tutorial, we will see how to convert an array of ints or numbers to a string in Go.
Below is the program for the same.
Program
Here is the program for the same.
package main
import (
"fmt"
"strconv"
)
func main() {
sample := []int{2, 1, 3}
output := ""
for _, v := range sample {
temp := strconv.Itoa(v)
output = output + temp
}
fmt.Println(output)
}
Output
213
Note: Check out our Golang Advanced Tutorial. The tutorials in this series are elaborative and we have tried to cover all conc ..read more
Golang By Example
1y ago
Overview
A simple regex can be used to check if a string contains single or multiple white spaces in Go.
Here is the program for the same
Program
package main
import (
"fmt"
"regexp"
)
func main() {
//Single whitespace
sampleWord := "Good morning"
isWhitespacePresent := regexp.MustCompile(`\s`).MatchString(sampleWord)
fmt.Println(isWhitespacePresent)
//Multiple Whitespace
sampleWord = "Good morning"
isMultipleWhitespacePresent := regexp.MustCompile(`\s*`).MatchString(sampleWord)
fmt.Println(isMultipleWhitesp ..read more
Golang By Example
1y ago
Overview
Below is a simple way to initialize or create a string in Go. In the below program, we have simply declared as well as defined a string named sample
Notice the syntax
sample := "test"
Here is the full program
Program
package main
import "fmt"
func main() {
sample := "test"
fmt.Println(sample)
}
Output
test
If we want to only declare a string variable then below is the way. A declared string is initialized to an empty string
package main
import "fmt"
func main() {
var sample string
fmt.Println(sample)
}
Output
Note: Check out our Golang Advanced Tuto ..read more
Golang By Example
1y ago
Overview
Enum in Golang can be created by using IOTA. Please refer to this post to learn more about IOTA
IOTA in Go (Golang)
In this post, we will see how to convert an IOTA or enum to a string value. By default when you will print an IOTA or enum value it will print the integer part of it. See this example. Later we will see an example of we can define a custom toString method to print the string value of IOTA or enum
Example
package main
import "fmt"
type Size uint8
const (
small Size = iota
medium
large
extraLarge
)
func main() {
fmt.Println(sm ..read more
Golang By Example
1y ago
Overview
IOTA provides an automated way to create an enum in Golang. Let’s see an example.
Example
package main
import "fmt"
type Size uint8
const (
small Size = iota
medium
large
extraLarge
)
func main() {
fmt.Println(small)
fmt.Println(medium)
fmt.Println(large)
fmt.Println(extraLarge)
}
Output
0
1
2
3
In above program we created a new type
type Size uint8
Then we declared some const of type Size. The first constant small is set to iota so it will be set to zero
small Size = iota
That’s why
fmt.Println(small ..read more
Golang By Example
1y ago
Sort a slice in Ascending order
sort.Ints package of going can be used to sort a full slice or a part of the slice. It is going to sort the string into Ascending order.
Below is the signature of the method
https://pkg.go.dev/sort#Ints
func Ints(x []int)
It takes a slice ‘x’ as an argument and sorts the slice ‘x’ in place.
Below is the program for the same
package main
import (
"fmt"
"sort"
)
func main() {
nums := []int{3, 4, 2, 1}
fmt.Printf("Before: %v", nums)
sort.Ints(nums)
fmt.Printf("\nAfter: %v", nums)
}
Output
Before: [3 4 2 1]
Aft ..read more
Golang By Example
1y ago
Overview
strings.Repeat method can be used to repeat a string multiple times in Go (Golang)
Here is the link to this function in the Go strings package
https://pkg.go.dev/strings#Repeat
Here is the signature of the method
func Repeat(s string, count int) string
The first argument is the original string and count is the number of times the strings needs to be repeated
Program
Here is the program for the same
package main
import (
"fmt"
"strings"
)
func main() {
copy := strings.Repeat("a", 2)
fmt.Println(copy)
copy = strings.Repeat("abc", 3 ..read more