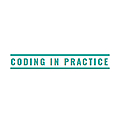
Coding in Practice
78 FOLLOWERS
We are a group of programmers who likes sharing our learnings. This site is only for learning purposes and contributors keep posting if they find something useful and that can be helpful for others also.
Coding in Practice
3y ago
Adapter pattern is a structural design pattern which works as a bridge between two incompatible interfaces. Here one should note that interfaces may be incompatible but their inner functionality should match the requirement. In this pattern client invokes adapter and adapter converts the request compatible to actual interface, in same way adapter converts response also compatible for client. In daily life we come across many adapter examples, like- adapter for mobile charger, card reader etc.
When to use adapter pattern
Use adapter design pattern when two interfaces are incompatible but having ..read more
Coding in Practice
3y ago
Object pool pattern is creational design pattern which keeps a set of already initialized objects to use whenever required, rather than creating a new instance each time. Object pool pattern is helpful when cost of object creation is high and they are required in large number. Basically object pool is a container that keeps some already created and initialized objects. Whenever object is required, then no need to create it but get it from object pool. After using the object, put it back in pool. Object pool pattern is having object scope.
When to use object pool pattern
Object pool pattern com ..read more
Coding in Practice
3y ago
In day to day programming, we all come across the scenarios where we need to create POJO classes. POJO classes have data members and their getters and setters and few additional methods like- hashCode(), equals(), toString() etc. You might have noticed that all these methods can be generated automatically using IDEs, means these methods are so predictable and adding them to class is like adding boilerplate code.
In Java-14, record type is introduced as preview feature which is similar like POJO classes. Records in java can be used to hold pure data and also can avoid boilerplate code. Let’s un ..read more
Coding in Practice
3y ago
Java 15 introduced sealed classes and interfaces as preview feature. Preview language features are complete and ready for developer’s use but can be modified in future as per developer’s feedback. Sealed classes and interfaces are developed to put restrictions on hierarchy. Let’s understand first about sealed classes.
Sealed classes
By defining a class as sealed class, we can put extra restrictions on class hierarchies. Inheritance is one of the main pillar OOP but also has some drawbacks. Inheritance makes base and child classes tightly coupled. Another problem is fragile base class. If base ..read more
Coding in Practice
3y ago
In day to day programming we get scenarios to create exact copy of an object. It helps us to reduce the boilerplate code and also reduces the complexity of object creation. Java provides us feature named “cloning” to copy the object. “Object” class in java provides clone() method to create copy of an object. To implement clone() method, java class needs to implement “Cloneable” interface which is a marker interface. Here one thing comes in mind that instead of using clone we can assign reference variable to another variable, like below-
Class1 obj1= new Class1();
Class1 obj2= obj1;
But it is c ..read more
Coding in Practice
3y ago
In day to day software implementation we come across situations where object creation is costly operation in terms of time and resources, creating database connection is one of such example. Hence creating new instance each time for such objects is not a wise decision. One possible way to overcome this issues is singleton pattern but in singleton pattern only one instance will be created and hence each client will be having the same state. It is not practical every time because sometimes for same object client may need different states. Here prototype pattern is best alternative.
Prototype pat ..read more
Coding in Practice
3y ago
Builder pattern separates the creation of object from its representation and same construction process can create different representations. It provides a way to construct object step by step and final step returns the object. But this pattern is not only restricted to the creation of complex objects but can also be used at other scenarios which we are going to discuss in this article. Since it is associated with creation of object hence it is a creational pattern and has object scope.
When to use builder pattern
Builder pattern can be used in below scenarios-
Object construction is complex ..read more
Coding in Practice
3y ago
Abstract factory design pattern is a creational design pattern sometimes also called as factory of factories or super factory. Let’s understand the definition of this pattern first-
“Provide an interface for creating families of related or dependent objects without specifying their concrete classes.”
So as per definition, abstract factory pattern is responsible to create family of related or dependent object and usually these objects are supposed to be used together. Hence abstract factory pattern adds one extra layer of abstraction that’s why it is also called factory of factories or super fa ..read more
Coding in Practice
3y ago
Factory design pattern is creational design pattern and used widely as it reduces the complexity of the code and helps to maintain loose coupling. In factory design pattern we create object without exposing creational logic or implementation class to the client. Client only deals with interface or abstract class. Factory pattern has class scope because this pattern revolve around the class types whose object needs to be sent back to the client and object sent to the client belongs to same interface or parent class. Also we are not performing any manipulation or restriction to the object ..read more
Coding in Practice
3y ago
Singleton pattern is creational design pattern that is used to restrict the instantiation of the class so that only single object for a class can be created. To access that object, single global point is exposed. Singleton is also most controversial design pattern as there are plenty of ways to break it and it is difficult to get tested, so some experts keep it in category of anti pattern and suggest to avoid it. We are going to discuss all these points in this article.
Singleton design pattern is having object scope because more than class we are concerned about object. How to restrict to sin ..read more