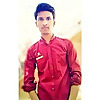
CodingWithRohit
101 FOLLOWERS
Welcome to codingwithrohit, This is one of the best sources to learn to program. We're dedicated to giving you the very best tutorial of programming language. Founded in 2020 by Rohit Srivastava, This is the beginning of our journey. From this website, many students learn programming languages all over the world and are thrilled that we're able to turn our passion into my own website.
CodingWithRohit
4y ago
The two sum problem is a common interview question, and it is a variation of the subset sum problem.
Given a list of numbers and a number k, return whether any two numbers from the list add up to k.
For example, given [10, 15, 3, 7] and k of 17, return true since 10 + 7 is 17.
Naive solutionA naive approach to this problem from google would be to loop through each number and then loop again through the array looking for a pair that sums to S. The running time for the below solution would be O(n2) because in the worst case we are looping through the array twice to find a pair.
a = [10,15 ..read more
CodingWithRohit
4y ago
Python is a programming language that is easy to learn and a powerful programming language. It has efficient high-level data structures and a simple but effective approach to object-oriented programming.
Python’s elegant syntax and dynamic typing, together with its interpreted nature, make it an ideal language for scripting and rapid application development in many areas on most platforms.
The Python interpreter and the extensive standard library are freely available in source or binary form for all major platforms from the Python Web site, https://www.python.org/, and may be fre ..read more
CodingWithRohit
4y ago
Array of Structures
We can declare an array of structures each element of an array is of structure type. An array of structures can be declared as
struct book b[10];
Here b[10] is an array of l0 elements, each of which is a structure of type struct student, means each element of b has 3 members, which are name, roll_no, and marks. These structures can be accessed through subscript notation. To access the individual members of these structures we'll use the dot tor as usual
Program to demon ..read more
CodingWithRohit
4y ago
The structure can be passed as arguments to functions in different ways. We can pass individual members, whole structure variable or structure pointers to the function.
Similarly, a function can return either a structure member or a whole structure variable or a pointer to a structure.
Passing Structure Members As Arguments
IN C, we can pass individual structure members as arguments to functions like any other ordinary variable.
#include<stdio.h>
void display(char name[],char author[]); struct website { char name[25] ; char author[25] ; }; main( ) { struct website b1={ "Co ..read more
CodingWithRohit
4y ago
In this tutorial we will teach you how to dynamically allocate memory in your C program using standard library functions:
malloc()
calloc()
free()
realloc()
These functions can be found in the <stdlib.h> header file.
1. malloc()
This function is used to allocate memory dynamically. The argument size specifies the number of bytes to be allocated. The type size_t is defined in stdlib.h as unsigned int. On success, malloc( ) returns a pointer to the first byte ( )f allocated memory. The returned pointer is of type void, which can be typecast to the appropriate type of pointer ..read more
CodingWithRohit
4y ago
In C programming, we can pass the arguments to the functions in two ways:
call by value
call by reference
In call by value, only the values of the argument are sent to the function and changes made to formal arguments and there is no change in the actual argument whereas.
In call by reference, addresses of arguments are sent to the functions, and changes made to the formal arguments change the actual arguments also, we can simulate call by reference with the help of pointer.
#include <stdio.h>
void swap(int *a, int *b);
int main()
{
a=5,b=8;
printf("Value before fu ..read more
CodingWithRohit
4y ago
In this tutorial, we will teach you about the relationship between arrays and pointers in C programming.
Before you move forward to learn about the relationship between arrays and pointers, please make sure to check these two topics:
C Arrays
C Pointer
We can also declare a pointer that can point to the whole array instead of the only one element of the array. This type of pointer is useful when talking about multidimensional arrays. Now we'll see how to declare a pointer to an array.
int (*ptr)[10];
In the above examples, ptr is a pointer that points to an array of 10 integers ..read more
CodingWithRohit
4y ago
We will teach you how to write C programs with the help of Functions, you will also find some examples of C programs to enhance your knowledge of functions.
In c programming, A function is a subprogram that is meant to perform some specific task.
To understand these examples in this page, you should have the knowledge of the following topics:
User-Defined Function
Types of User-defined functions
Scope of a local variable
1. Write a c program to find the square of the given number
#include<stdio.h>
// function prototype, also called function declaration
float square ( float z );&n ..read more
CodingWithRohit
4y ago
Write a C program to print an array #include <stdio.h>
int main() {
int array[10] = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100}; //array initialize
int i;
for(i = 0; i < 10; i++)
printf("%d ", array[i]);
return 0;
}
Output
10 20 30 40 50 60 70 80 90 100
Write a C Program to print an array in reverse order #include <stdio.h>
int main() {
int array[10] = {10, 20, 30, 40, 50, 60, 70, 80, 90, 100}; //array initialize
int i;
for(i = 9 ..read more
CodingWithRohit
4y ago
A scope refer to a region of the program, and the scope of variables is the region of the program where the variables can be accessed after its declaration.
In C programming, we can declared variable in three ways. These are:
Types of variable
Position and scope
1. local variables
These types of variable we declare Inside a function or a block. They are only accessible to their function or block where they declare.
2. Formal parameters
Formal Parameters arefer those parameters which are used in the function parameters.
3. Global variables
These types of variable are defined outside ..read more