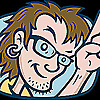
David Walsh
14,186 FOLLOWERS
A blog featuring tutorials about JavaScript, HTML5, AJAX, PHP, CSS, WordPress, and everything else development. David Walsh is Mozilla's senior web developer and the core developer for the MooTools Javascript Framework.
David Walsh
1w ago
This past weekend I had the opportunity to be what every father wants, if only for a moment: the “cool dad”. My wife was out of town and my youngest son wanted to play PUBG. I caved in, taught him the basic FPS key binds, and he was having a great time. While he was fragging out, he pressed a bunch of random keys and ended up changing movement buttons. Suddenly the traditional WASD movement keys were useless and the arrow keys triggered movement.
Of course, this was a degradation of player experience. After struggling to figure out what my son did, I found the solution.
To restore the WASD key ..read more
David Walsh
3w ago
Modals have been an important part of websites for two decades. Stacking contents and using fetch to accomplish tasks are a great way to improve UX on both desktop and mobile. Unfortunately most developers don’t know that the HTML and JavaScript specs have implemented a native modal system via the popover attribute — let’s check it out!
The HTML
Creating a native HTML modal consists of using the popovertarget attribute as the trigger and the popover attribute, paired with an id, to identify the content element:
<!--
"popovertarget" attribute will map to "id" of popover contents
--> ..read more
David Walsh
1M ago
AI media creation has expanded to incredible video art and a host of other important improvements, and LimeWire is leading the way in creating an awesome interface for the average user to become an AI artist. Limewire has just released its Developer API, a method for engineers like us to create dynamic AI art on the fly!
Quick Hits
Free to sign up!
Provides methods to create a variety of quality images from any number of AI services and algorithms
Create images based on text and other images
Modify existing images to scale them, remove backgrounds, and more
Use JavaScript, PHP, Python, or an ..read more
David Walsh
3M ago
Anyone is capable of having their caps lock key on at any given time without realizing so. Users can easily spot unwanted caps lock when typing in most inputs, but when using a password input, the problem isn’t so obvious. That leads to the user’s password being incorrect, which is an annoyance. Ideally developers could let the user know their caps lock key is activated.
To detect if a user has their keyboard’s caps lock turn on, we’ll employ KeyboardEvent‘s getModifierState method:
document.querySelector('input[type=password]').addEventListener('keyup', function (keyboardEvent) {
const c ..read more
David Walsh
3M ago
One of the HTML elements that frequently comes into collision with CSS is the img element. As we learned in Request Metrics’ Fixing Cumulative Layout Shift Problems on DavidWalshBlog article, providing image dimensions within the image tag will help to improve your website’s score. But in a world where responsive design is king, we need CSS and HTML to work together.
Most responsive design style adjustments are done via max-width values, but when you provide a height value to your image, you can get a distorted image. The goal should always be a display images in relative dimensions. So how do ..read more
David Walsh
3M ago
Over 50 thousand developers visit DavidWalshBlog every month from around the world to learn JavaScript tricks and fix problems in their code. Unfortunately, some of them have a slow experience on the site.
David tracks the performance of his Core Web Vitals and overall performance with Request Metrics. Recently, we noticed that his CLS performance score was trending pretty slow for both desktop and mobile users.
Wait, what is CLS?
Cumulative Layout Shift (CLS) is one of the Core Web Vital performance metrics. It doesn’t measure load time directly, instead it measures how much a page shifts whi ..read more
David Walsh
4M ago
Ask any software engineer and they’ll tell you that coding date logic can be a nightmare. Developers need to consider timezones, weird date defaults, and platform-specific date formats. The easiest way to work with dates is to reduce the date to the most simple format possible — usually a timestamp. To get the immediate time in integer format, you can use Date.now:
const now = Date.now(); // 1705190738870
I will oftentimes employ Date.now() in my console.log statements to differentiate likewise console.log results from each other. You could also use that date as a unique identifier for an ev ..read more
David Walsh
4M ago
User input from HTML form fields is generally provided to JavaScript as a string. We’ve lived with that fact for decades but sometimes developers need to extract numbers from that string. There are multiple ways to get those numbers but let’s rely on regular expressions to extract those numbers!
To employ a regular expression to get a number within a string, we can use \d+:
const string = "x12345david";
const [match] = string.match(/(\d+)/);
match; // 12345
Regular expressions are capable of really powerful operations within JavaScript; this practice is one of the easier operations. Converti ..read more
David Walsh
4M ago
Streaming services have revolutionized content delivery, sending linear media companies into a panic as they watch traditional cable services decay. “Cutting the cord” is a common practice these days, but the streaming landscape isn’t perfect. We’re a decade into streaming so I wanted to share my thoughts on the state of new media: first impressions, second thoughts, and the third degree!
Netflix is king thanks to having first mover advantage, and making smart financial moves over the past six months, but Netflix’s content is unremarkable. Their recent wins are USA’s Suits and content licens ..read more
David Walsh
5M ago
As the demands of the web change and developers experiment with different user experiences, the need for more native language improvements expands. Our presentation layer, CSS, has done incredibly well in improving capabilities, even if sometimes too slow. The need for native support for automatically expanding textarea elements has been long known…and it’s finally here!
To allow textarea elements to grow vertically and horizontally, add the field-sizing property with a value of content:
textarea {
field-sizing: content; // default is `fixed`
}
The default value for field-sizing is fixed ..read more