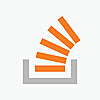
Stack Overflow » Algorithm
90 FOLLOWERS
Read the most recent 30 Algorithm posts from Stack Overflow. The world's largest online community for developers, helping write the script of the future by serving developers and all technologists.
Stack Overflow » Algorithm
17h ago
I'm trying to implement a function that populates the n-ary tree with these top two priorities (in this order): 1. Insert at the maximum possible depth. 2. Insert from left to right, as such an example is a depth of 3 and 12 elements with n of 3 children in the image:
n-ary tree example
This is my attempt so far, I have already tried to populate up until the maximum possible depth but got me confused on how I could implement the left to right constraint
int Tree::insertcell(int data, int depth, int* done, int maxchild, TreeNode* node) {
for(int i = 0; i < maxchild; i++){
cout ..read more
Stack Overflow » Algorithm
17h ago
Is Dijkstra's algorithm the most suitable for finding the shortest distance between two nodes, where all of the paths in the graph are equal to 1.
If not what is a more time efficient way of implementing this ..read more
Stack Overflow » Algorithm
17h ago
As I opted for option 1 and 3 (-6, infinity),(-7, Infinity) and applied the Bellman-Ford algorithm for shortest path calculation, the calculation did not show any negative cycles after n’th steps for both the options(I might be wrong). Therefore is it valid to say that the value of “X” should be at least “-7” to avoid any negative cycles ..read more
Stack Overflow » Algorithm
17h ago
I am unable to understand the complete algorithm of step by step in prims algorithm
I am unable to get the concept of prims algorithm. I am unable to dry run a problem from prims algorithm. Plessis provide me a complete explanation of prims algorithm ..read more
Stack Overflow » Algorithm
17h ago
I have a dataset of observations that come from the interaction of two elements.
Imagine that we have Bees and Flowers and a certain value associated with each of their observed interactions.
This data is saved in a long format like the following
Bee
Flower
Value
A
X
...
A
Z
...
B
X
...
B
Y
...
C
Y
...
C
Z
...
I want to split some pairs of Bee-Flower and put them in a separate 'test' set, but I also want to make sure that the Bee and the Flower can be found in the 'original' set.
By that I mean that if the pair AX is found in the test set, then there must be at least one ..read more
Stack Overflow » Algorithm
17h ago
I have some list of expressions,
const expressions = [
'A&(B|C)',
'A|(B&C)',
'A|(B&(C|D))',
'A|(B&C&D)',
];
Out of this, I need to get an output like,
[[A, B], [A, C]]
[[A], [B, C]]
[A, [B, C], [B, D]]
[A, [B, C, D]]
I tried to do this in the below way, But getting an empty arrays instead of correct result.
class ParsedExpression {
operator: string | null;
variables: string[];
subExpressions: ParsedExpression[];
constructor(operator: string | null, variables: string[], subExpressions: ParsedExpression[]) {
this.operator = opera ..read more
Stack Overflow » Algorithm
17h ago
Building a system which can identify all users affected by system failure & triggers Push notifications(PN) once systems are back up, either for all users of app opens OR specific page say for an e-commerce - CART/ADDRESS/PAYMENT/PRODUCT LISTING PAGE, failing for certain time & users were not able to do things served by these pages/micro-services.
We want to know what's the best to identify affected users and save them in a data-store for say a day or two, and then we can make the range query in that internal, to read all those users and send them failure fixed communication.
System ou ..read more
Stack Overflow » Algorithm
17h ago
I have an array of size 256 (index ranging [0, 255]), where each array element is an 8 byte integer. Now I am randomly generating the indexes of this array and incrementing the values stored at that index. After the end of the trials, the indexes that still have value 0 would be considered as memory wastage. For this I believe the average memory loss will be 128x8 bytes (on an average the array would be half filled). Is my understanding correct?
Now if I divide the array into 4 sub arrays of 64 elements each (call it subarray 1-4). The index ranges for these subarray would be [0,63] subarray1 ..read more
Stack Overflow » Algorithm
17h ago
I have to create code for a UI in Python. I basically have 4 options, and each option, of course, will print a result desired. The code is below. I am missing a print line for one, but I asked my instructor about that. What I need to know as well- how can I test my UI code to see if I will get the results I am wanting?
getPackageStatus = True
while getPackageStatus:
# Options for UI
print("\nWhat Would You Like to Check Today?")
print("1. All package statuses and Total Truck mileage")
print("2. Status of all Packages at a given time")
print("3. Single package status with a ..read more
Stack Overflow » Algorithm
17h ago
I have to implement algorithm which solves following problem:
Inputs:
A list of up to 300 points in the plane, with integer coordinates.
A list of distances between every pair of points, which are Manhattan distances.
A score of at least 1 for every point
Output: A path through at least half of all points, which maximizes the ratio of (sum of scores) / (sum of distances) along the path, and which never passes the same point twice. Note that we can accept a good solution over the best solution if that buys us a significantly better running time.
Thoughts:
Differences between classic traveller ..read more